Yesterday I made a mistake: I accepted an invitation to the organization on GitHub, which had thousands of active repositories. And, because my Notification Settings had “Automatically watch repositories” checked, I started to receive hundreds of emails.
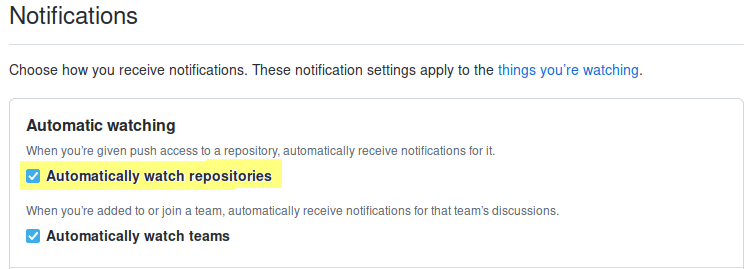
Obviously, it was a tedious task to go to the Watching page and manually unwatch those thousands of repositories. I decided to write a simple script in Node.js — in the hope that someone might find it useful.
First of all, you need to go to GitHub’s Developer Settings and generate an access token. I granted my token the notifications
and repo
permissions; probably, notifications
alone would be enough, but I didn’t check that.
Then, you will need to create a project and install the dependencies (only one):
npm init -y npm i @octokit/core
Here goes the script:
const { Octokit } = require("@octokit/core"); const octokit = new Octokit({ auth: 'the generated access token goes here' }); (async () => { let page = 1; let subs; let repos = []; do { subs = await octokit.request('GET /user/subscriptions', { per_page: 100, page, }); repos = repos.concat( subs.data .filter((sub) => sub.owner.login === 'orgname') .map((sub) => [sub.owner.login, sub.name])) ; ++page; } while (subs.data.length > 0); for (const [owner, repo] of repos) { console.log(owner + '/' + repo); await octokit.request('DELETE /repos/{owner}/{repo}/subscription', { owner, repo }); } })();
The logic is pretty straightforward: in the do/while
loop, we collect the repositories we want to unsubscribe from, and in the for
loop, we unsubscribe from them.
On the line 17 (.filter((sub) => sub.owner.login === 'orgname')
), we implement the logic to determine whether we need to unsubscribe from the repository. In this example, we unsubscribe from all repositories in the orgname
organization. The organization name will be in sub.owner.login
; the repository name will be in sub.name
, the full name (organization plus repository) will be sub.full_name
.
Run the script: node index.js
and enjoy!